Overview
The ampliFi SDK is a private Javascript framework for internet-banking, mobile-banking, or any other banking applications. This SDK is a powerful starter pack with which you can quickly build new banking applications or add banking services into existing applications. This framework creates a developer-friendly abstraction layer that is pre-integrated with all of our ampliFi API and can be used in mobile and/or web browser environments, as well as in server-side node.js environments. Thus, it not only creates a gateway to our payment rails and services available through connectFi, such as ACH, Bill Payment, Wires, Card Issuing, and Acquiring, but it also provides access to ampliFi's middleware features - including User Management, Authentication, Velocity Limits, Imaging, and other microservices vital to banking applications. The ampliFi SDK is a versatile and customizable tool that will allow you to build financial applications that work for your business.
Illustration
The ampliFi SDK framework is part of a larger software suite. The architecture diagram below illustrates the various layers of this framework and how they relate to your business and other services. There are several entry points through which you can integrate the ampliFi BaaS solutions that your business needs into your application.
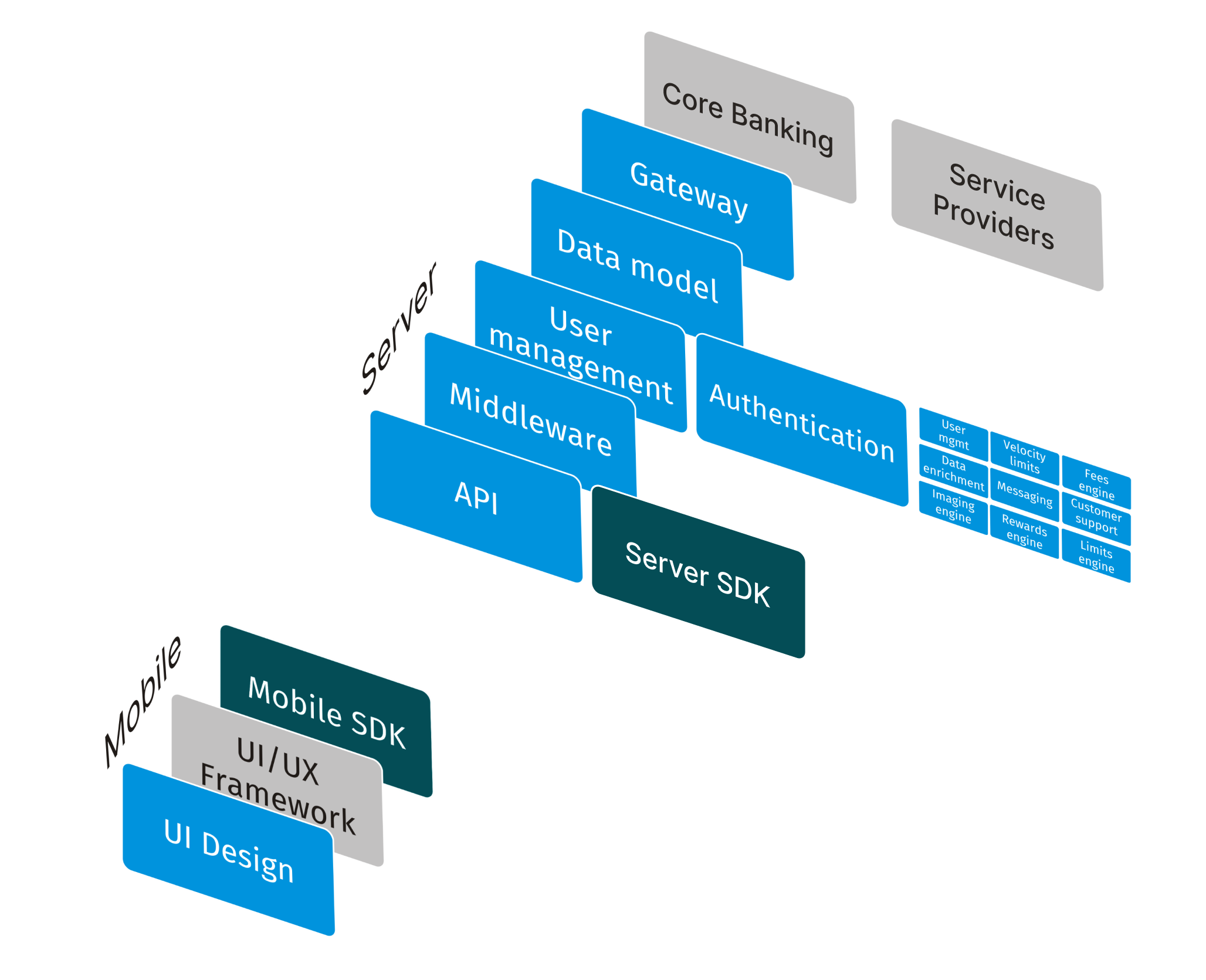
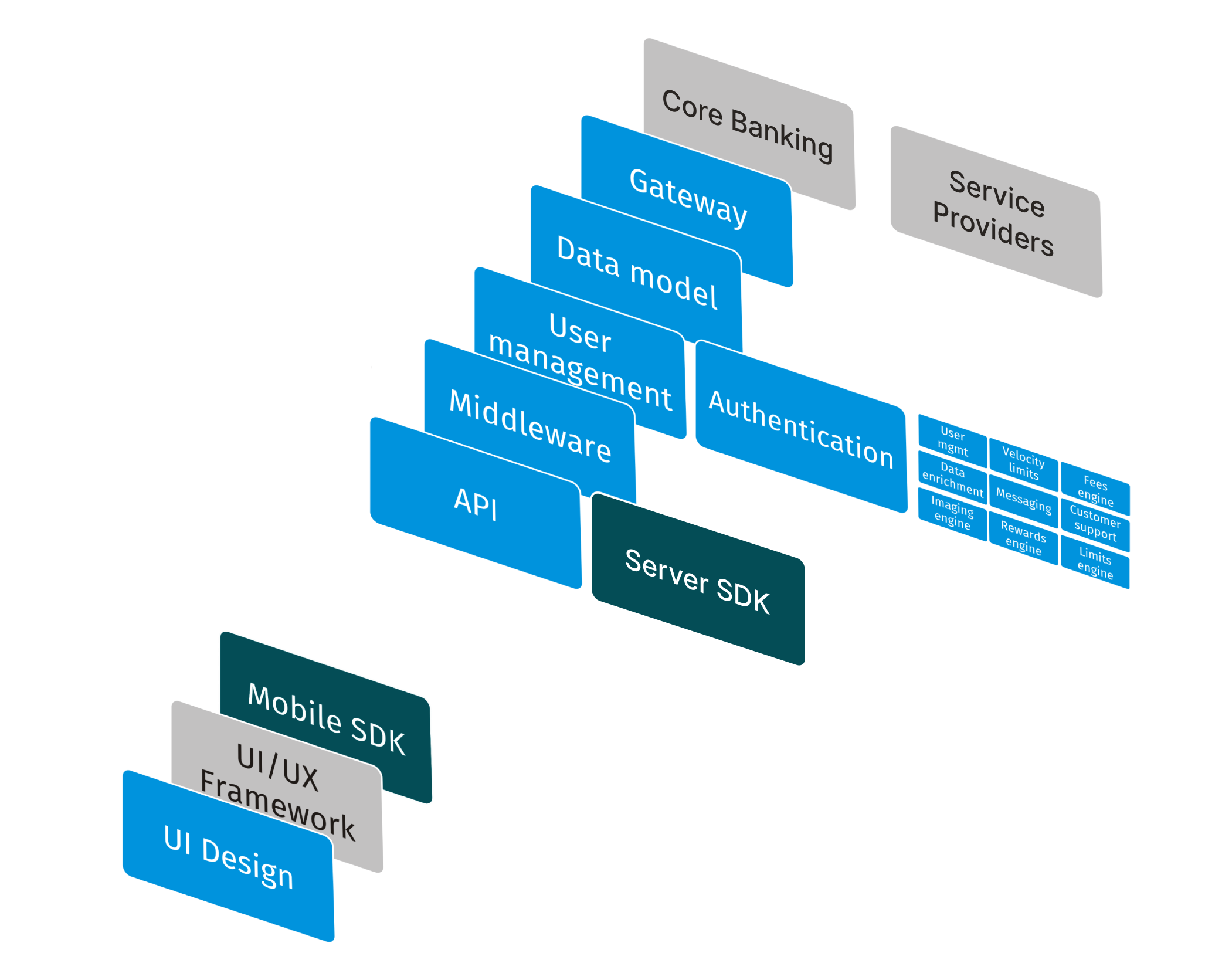
Beginning with the foremost layer in the diagram, a user interface (UI Design layer) can be built from scratch using our ampliFi UI Kit with React, or you can use an existing UI to interact with the ampliFi software suite. The ampliFi SDK can then be leveraged for fast client-side application development targeting mobile devices and/or web browsers. See our Getting Started > Quick Start guide for an example walkthrough showing how to use the ampliFi SDK in the browser to listen for, create, and react on browser events using the following class calls to TheM (The "M").
TheM.addSubscribers({ //add a listener (subscriber)
name: "test_myNewEvent", //"test_" is the default LOCAL_STORAGE_TAG_PREFIX. This can be customized using config.LOCAL_STORAGE_TAG_PREFIX when initializing TheM.
fn: (givenEventName, payload) => {
if (givenEventName === "test_myNewEvent" ) {
console.log(`This is the payload: ${JSON.stringify(payload)}`);
}
}
});
TheM.callSubscribers("test_myNewEvent", {
key1: "value1",
key2: "value2"
}
) //call the listener (subscriber).
The ampliFi SDK is also a powerful tool for server-side development, allowing the SDK to operate at two different layers in this architecture. This empowers your app with the flexibility to employ similar functionality on either the client-side or server-side, depending on your use case. The example calls above can be implemented in a node.js environment just as easily as a browser environment.
When using the following alternative method for listening to and reacting on events, the SDK will check whether the current environment is node.js or a browser and will function accordingly.
TheM.on("myNewEvent", (given) => { //add a listener (subscriber)
console.log(given); //This will log the CustomEvent
if (given.detail) {
console.log(given.detail); //given.detail will contain whatever object or value is passed into the event function as a payload when TheM.newEvent(givenEventName, payload) is called
}
}, true);
//This will call the "myNewEvent" listener that was added using TheM.on, as well as any subscribers in TheM.subscribers that are prefixed with the LOCAL_STORAGE_TAG_PREFIX, such as "test_myNewEvent".
TheM.newEvent("myNewEvent", {key1: "value1", key2: "The event was fired", key3: 42});
In addition, the server-side node.js environment is where you can integrate and interact with the ampliFi core. The ampliFi core modules (or middleware) are accessed through restful API endpoints and/or the ampliFi SDK. These middleware modules provide the ability to create complex, custom business logic such as developing segment- or product- specific generic requests, defining card restrictions, creating custom onboarding experiences for prospective users depending on the type of segment, and more. The ampliFi core modules also provide the layer of server-side functionality shown that includes user management, authentication, messaging, limits, etc.
The next architecture layer depicts the data model, which consists of the back-end database storage as well as the logic used when interacting with the database and beyond.
The penultimate layer in this diagram shows our connectFi API. For more information on connectFi, including the various payment rails that are available, see the connectFi documentation. Finally, the last layer in the architecture diagram represents the core banking and other services that we partner with.
Key Features
The ampliFi SDK incorporates all of the key features of the ampliFi core (explained in the OVERVIEW > Introduction section of this documentation), as well as the following additional features:
-
Local data caching -- The ampliFi SDK framework maintains a local copy of the customer's data and synchronizes it with the server as needed. Storing all data locally allows for a super fast UI experience because there are no unnecessary server requests. Repeat requests are served from in-memory cache, thus saving traffic and making mobile and web apps much more responsive.
-
Offline functionality -- The SDK framework can function offline, which is vital for mobile apps: objects can be stored to and fetched from persistent storage on the device.
-
Client-side validation -- Our ampliFi SDK also supports client-side validation, so client-side applications can display errors even before a request is sent to the server.
-
Versatile -- This framework plays well with other front-end frameworks such as ReactJS, VueJS, Svelte, etc. It is functional in browsers and in node, so it can be used in chatbots, IVR, USSD, and even ATMs.
-
Designer- and Developer-friendly -- The ampliFi SDK provides easy access to all of the data, objects, methods, and banking functionality components necessary to build in app financial services through a single class, called
TheM
. Development of the front-end and the back-end can be segregated and continue in parallel. Thus, front-end designers can construct the UI and never have to worry about complexities such as cross-currency foreign funds transfers. -
Automatic data updates -- Our framework will automatically take care of fetching all of the most up-to-date account information from each of the back-offices with whom a user has accounts. A call will first return the locally stored data, then it will fetch the fresh data from the server, and will lastly let the front end know that the data needs to be re-rendered. It will serve all other calls from local storage until the data has updated. Server requests do not block the UI as the requests happen in the background: all requests are pooled, data is cached in memory, and data can be saved in local storage for persistence and offline access.
The ampliFi SDK framework is best described as part of the "M" in the MVC (Model-View-Controller) approach to application architecture.
Extending and Re-using the Framework
The ampliFi SDK framework was designed to be extendable, so you can easily add new functionality and re-use all of the infrastructural code which securely stores the data on the device, authenticates the user, communicates with the server, etc. If you need a new kind of account, you can extend the basic account class and add the desired functionality. Need a Zelle payee? Extend the basic payee class and use the Paypal payee as a guideline.
Demo
Once you have spoken with a support representative and received access to an ampliFi sandbox instance to work with, then see the Quick Start guide in the Getting Started section of this this documentation for instructions on how to install and start working with the ampliFi SDK.